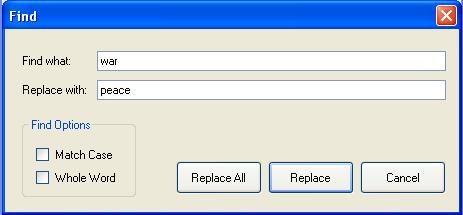
Introduction
This article describes how to create a find and
replace method for the RichTextBox control. It is
easy to understand. If you develop a word processing
application this method will be a grate gadget for you. You
can create any method for any control like this.
How To Create It
1. First, create a new project and name it
"FRRichTextBox"
2. Then we need to create a class
RichTextBoxFR.vb. to
create it, click on Add New Item
button on the Standard Toolbar.
3. Select Class
from the template and name it
RichTextBoxFR.vb and Click on
Add button.
4. We use inherits statement and
derive our class from RichTextBox
Class
Public Class RichTextBoxFR
Inherits RichTextBox
End Class
5.
We create a
FindAndReplace
method with two arguments -
FindText
and ReplaceText.
Public Class RichTextBoxFR
Inherits RichTextBox
Public Sub FindAndReplace(ByVal FindText As String, ByVal ReplaceText As String)
Me.Find(FindText)
If Not Me.SelectionLength = 0 Then
Me.SelectedText = ReplaceText
Else
MsgBox("The following specified text was not found: " & FindText)
End If
End Sub
End Class
Me here is
RichTextBox.
We use
Me.Find(FindText) to search
the text of the
RichTextBox
control and select the text. If the search text is found,
RichTextBox select it and
SelectionLength of RichTextBox
will be > 0. Then we use if else statement to replace that
text as above.
6. Now we create another
FindAndReplace method by
overloading our previous
FindAndReplace method. You can see that method
has five arguments -
FindText,
ReplaceText,
ReplaceAll, MatchCase
and
WholeWord
Collapse
Public Class RichTextBoxFR
Inherits RichTextBox
Public Sub FindAndReplace(ByVal FindText As String, ByVal ReplaceText As String)
Me.Find(FindText)
If Not Me.SelectionLength = 0 Then
Me.SelectedText = ReplaceText
Else
MsgBox("The following specified text was not found: " & FindText)
End If
End Sub
Public Sub FindAndReplace(ByVal FindText As String, ByVal ReplaceText As String, ByVal ReplaceAll As Boolean, _
ByVal MatchCase As Boolean, ByVal WholeWord As Boolean)
Select Case ReplaceAll
Case False
If MatchCase = True Then
If WholeWord = True Then
Me.Find(FindText, RichTextBoxFinds.MatchCase Or RichTextBoxFinds.WholeWord)
Else
Me.Find(FindText, RichTextBoxFinds.MatchCase)
End If
Else
If WholeWord = True Then
Me.Find(FindText, RichTextBoxFinds.WholeWord)
Else
Me.Find(FindText)
End If
End If
If Not Me.SelectionLength = 0 Then
Me.SelectedText = ReplaceText
Else
MsgBox("The following specified text was not found: " & FindText)
End If
Case True
Dim i As Integer
For i = 0 To Me.TextLength 'We know that strings we have to replace < TextLength of RichTextBox
If MatchCase = True Then
If WholeWord = True Then
Me.Find(FindText, RichTextBoxFinds.MatchCase Or RichTextBoxFinds.WholeWord)
Else
Me.Find(FindText, RichTextBoxFinds.MatchCase)
End If
Else
If WholeWord = True Then
Me.Find(FindText, RichTextBoxFinds.WholeWord)
Else
Me.Find(FindText)
End If
End If
If Not Me.SelectionLength = 0 Then
Me.SelectedText = ReplaceText
Else
MsgBox(i & " occurrence(s) replaced")
Exit For
End If
Next i
End Select
End Sub
End Class
7. We can use RichTextBoxFinds enumerations
(which enables us to specify how the search is performed
when the Find method is called)such as
MatchCase,
WholeWord, for advance search by thinking
logically.
8. When we use
ReplaceAll = True, we do not know how many
strings we have to replace, but we know that Strings we have
to replace < textlength of the richtextbox. We use For
Loop statement to implement it.
How To Use It
Now we have created our
RichTextBoxFR
control.
9. Select Build
Menu --> Build Solution
10. Select
RichTextBoxFR from the
ToolBox and draw it on the form.
11. Create a new form as above and name
it frmFind. This form
has
i. Two TextBoxes :-
FindTextBox and
ReplaceTextBox
ii. Three Buttons :-
btnReplaceAll,
btnReplace and
btnCancel
iii. Two CheckBoxes :-
MatchCase,
WholeWord
12. Here are the codes for the form
Collapse
Public Class frmFind
Private Sub btnReplaceAll_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnReplaceAll.Click
If MatchCase.Checked Then
If WholeWord.Checked Then
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, True, True, True)
Else
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, True, True, False)
End If
Else ' If FindMe.MatchCase.Checked = False
If WholeWord.Checked Then
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, True, False, True)
Else
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, True, False, False)
End If
End If 'End FindMe.MatchCase.Checked
End Sub
Private Sub btnReplace_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnReplace.Click
If MatchCase.Checked Then
If WholeWord.Checked Then
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, False, True, True)
Else
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, False, True, False)
End If
Else 'If FindMe.MatchCase.Checked = False
If WholeWord.Checked Then
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, False, False, True)
Else
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, False, False, False)
End If
End If 'End FindMe.MatchCase.Checked
End Sub
End Class
History
created - 17 March 2006